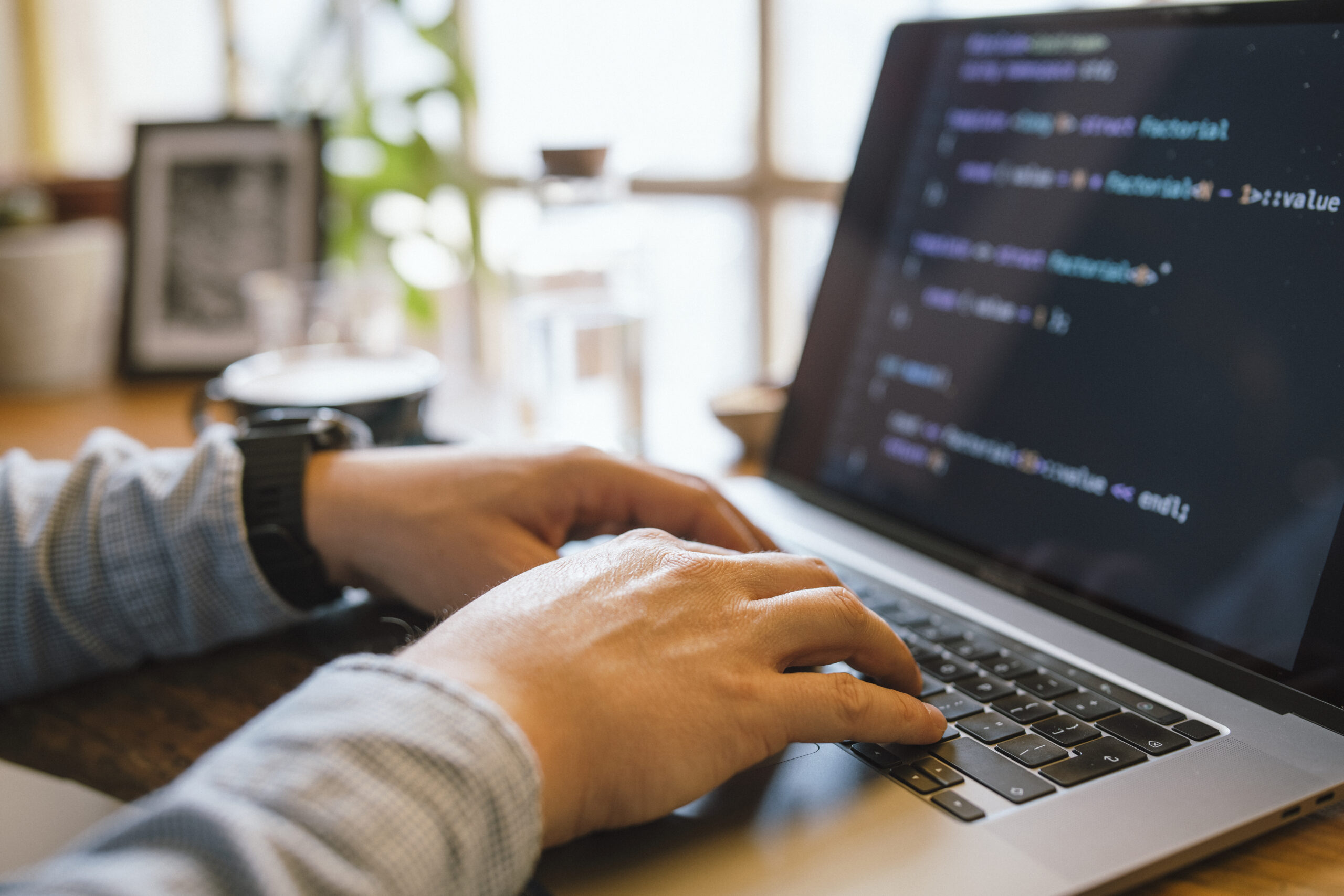
Debugging is Just about the most important — however typically missed — abilities inside a developer’s toolkit. It's actually not almost fixing broken code; it’s about comprehension how and why points go Completely wrong, and learning to Believe methodically to unravel challenges competently. Whether or not you're a newbie or perhaps a seasoned developer, sharpening your debugging techniques can help save several hours of annoyance and radically improve your productivity. Allow me to share many approaches to help you developers level up their debugging sport by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest ways builders can elevate their debugging techniques is by mastering the tools they use everyday. Though producing code is one particular Portion of improvement, knowing how you can interact with it correctly through execution is equally essential. Modern improvement environments occur Outfitted with potent debugging abilities — but a lot of developers only scratch the surface area of what these applications can perform.
Consider, such as, an Built-in Improvement Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, move by way of code line by line, and in many cases modify code within the fly. When made use of accurately, they let you notice particularly how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-stop developers. They assist you to inspect the DOM, check community requests, see authentic-time efficiency metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip discouraging UI problems into workable responsibilities.
For backend or technique-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about managing procedures and memory management. Understanding these applications may have a steeper Understanding curve but pays off when debugging general performance difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Model Manage techniques like Git to know code historical past, come across the precise moment bugs had been launched, and isolate problematic improvements.
In the end, mastering your equipment suggests likely beyond default options and shortcuts — it’s about establishing an personal knowledge of your improvement surroundings to ensure when difficulties crop up, you’re not shed in the dark. The higher you recognize your equipment, the greater time it is possible to shell out fixing the particular trouble rather then fumbling as a result of the procedure.
Reproduce the condition
One of the more significant — and often ignored — ways in helpful debugging is reproducing the problem. Prior to leaping into your code or creating guesses, developers have to have to produce a regular setting or situation exactly where the bug reliably appears. Without having reproducibility, fixing a bug results in being a activity of probability, usually leading to wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire queries like: What actions led to The difficulty? Which natural environment was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The greater depth you have, the a lot easier it gets to isolate the exact disorders beneath which the bug happens.
When you’ve gathered sufficient information, try and recreate the issue in your neighborhood natural environment. This could signify inputting the identical data, simulating related user interactions, or mimicking technique states. If The difficulty seems intermittently, think about producing automated assessments that replicate the edge situations or point out transitions included. These tests not merely assistance expose the trouble but will also avoid regressions Sooner or later.
Often, The difficulty could be natural environment-particular — it would transpire only on certain working systems, browsers, or beneath unique configurations. Using resources like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms might be instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a action — it’s a mindset. It demands persistence, observation, and also a methodical strategy. But when you finally can continuously recreate the bug, you're currently halfway to repairing it. By using a reproducible state of affairs, You should use your debugging resources a lot more properly, take a look at probable fixes properly, and connect much more Obviously along with your crew or end users. It turns an abstract grievance into a concrete problem — Which’s the place builders prosper.
Examine and Have an understanding of the Mistake Messages
Mistake messages are often the most valuable clues a developer has when some thing goes wrong. Rather than seeing them as frustrating interruptions, builders should really discover to treat error messages as immediate communications within the method. They often show you what precisely occurred, where it transpired, and from time to time even why it took place — if you know how to interpret them.
Begin by reading the information diligently As well as in complete. Several builders, specially when beneath time pressure, look at the main line and quickly commence making assumptions. But further inside the error stack or logs may well lie the correct root result in. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them 1st.
Break the mistake down into sections. Is it a syntax mistake, a runtime exception, or possibly a logic error? Will it point to a certain file and line quantity? What module or perform brought on it? These queries can guide your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and learning to recognize these can greatly hasten your debugging process.
Some problems are imprecise or generic, and in People conditions, it’s essential to look at the context wherein the error transpired. Look at associated log entries, enter values, and up to date changes inside the codebase.
Don’t ignore compiler or linter warnings both. These generally precede bigger challenges and provide hints about prospective bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Understanding to interpret them correctly turns chaos into clarity, aiding you pinpoint troubles a lot quicker, decrease debugging time, and become a far more successful and confident developer.
Use Logging Properly
Logging is one of the most potent resources in the developer’s debugging toolkit. When applied correctly, it offers serious-time insights into how an software behaves, aiding you recognize what’s occurring beneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic starts with knowing what to log and at what level. Popular logging levels include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in-depth diagnostic information during development, Facts for typical gatherings (like thriving get started-ups), Alert for opportunity difficulties that don’t split the appliance, ERROR for precise complications, and Deadly once the program can’t keep on.
Stay away from flooding your logs with extreme or irrelevant information. Too much logging can obscure vital messages and decelerate your program. Concentrate on important activities, state variations, enter/output values, and significant selection details with your code.
Format your log messages Plainly and continuously. Incorporate context, including timestamps, ask for IDs, and function names, so it’s easier to trace troubles in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
All through debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments wherever stepping via code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Using a well-imagined-out logging approach, it is possible to lessen the time it will take to identify challenges, obtain further visibility into your applications, and Enhance the Over-all maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not only a complex undertaking—it is a kind of investigation. To correctly determine and correct bugs, builders will have to method the method just like a detective fixing a secret. This frame of mind can help stop working complex concerns into manageable areas and abide by clues logically to uncover the foundation cause.
Begin by accumulating proof. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality difficulties. Identical to a detective surveys against the law scene, obtain as much related details as you'll be able to with no jumping to conclusions. Use logs, examination circumstances, and consumer reviews to piece with each other a transparent photo of what’s occurring.
Upcoming, sort hypotheses. Check with on your own: What may very well be resulting in this habits? Have any alterations not long ago been designed to your codebase? Has this situation transpired just before under similar instances? The target will be to slim down opportunities and recognize prospective culprits.
Then, check your theories systematically. Try to recreate the condition in a very controlled atmosphere. If you suspect a selected functionality or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code questions and Permit the outcomes lead you nearer to the truth.
Spend shut consideration to little aspects. Bugs generally conceal during the minimum expected destinations—like a missing semicolon, an off-by-just one error, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty without having absolutely comprehension it. Temporary fixes may perhaps conceal the real trouble, just for it to resurface afterwards.
Finally, retain notes on what you tried and discovered. Just as detectives log their investigations, documenting your debugging procedure can conserve time for foreseeable future challenges and aid Some others have an understanding of your reasoning.
By thinking similar to a detective, developers can sharpen their analytical abilities, method troubles methodically, and turn into simpler at uncovering hidden troubles in elaborate methods.
Publish Checks
Writing exams is one of the simplest ways to improve your debugging expertise and Total progress performance. Exams not merely enable capture bugs early but also serve as a safety net that offers you confidence when creating adjustments to the codebase. A properly-examined software is simpler to debug as it means that you can pinpoint accurately where by and when an issue happens.
Start with unit tests, which concentrate on person functions or modules. These little, isolated tests can quickly reveal regardless of whether a certain bit of logic is Functioning as anticipated. Whenever a check fails, you instantly know where to look, significantly lessening enough time expended debugging. Device assessments are Specifically helpful for catching regression bugs—problems that reappear after Beforehand staying mounted.
Up coming, integrate integration assessments and conclude-to-finish exams into your workflow. These help make sure several areas of your application do the job jointly easily. They’re particularly handy for catching bugs that arise in complicated units with various parts or solutions interacting. If a little something breaks, your exams can tell you which Element of the pipeline failed and less than what problems.
Writing checks also forces you to Imagine critically regarding your code. To test a aspect effectively, you need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge By natural means potential customers to better code framework and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the take a look at fails consistently, it is possible to deal with fixing the bug and look at your check move when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
Briefly, writing exams turns debugging from the disheartening guessing game into a structured and predictable system—assisting you catch far more bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s easy to become immersed in the trouble—observing your display for hrs, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and sometimes see The problem from a new standpoint.
If you're far too near the code for far too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable glitches or misreading code you wrote just several hours previously. In this particular point out, your Mind gets considerably less productive at difficulty-solving. A short wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your concentration. A lot of developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job during the qualifications.
Breaks also aid avoid burnout, Particularly during for a longer period debugging classes. Sitting in front of a display screen, mentally stuck, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do anything unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually brings about faster and simpler debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and rest is a component of resolving it.
Learn From Just about every Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can read more train you a thing valuable in case you go to the trouble to replicate and analyze what went Improper.
Start off by inquiring on your own a handful of key concerns once the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device tests, code reviews, or logging? The answers often reveal blind places in your workflow or understanding and assist you to Develop stronger coding routines moving ahead.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or retain a log in which you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring issues or popular issues—you can proactively keep away from.
In crew environments, sharing Everything you've discovered from a bug with all your friends could be Particularly effective. Whether or not it’s via a Slack concept, a short generate-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. In place of dreading bugs, you’ll commence appreciating them as essential areas of your improvement journey. In fact, a number of the best developers are not the ones who generate best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to the talent set. So following time you squash a bug, have a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging abilities can take time, observe, and persistence — even so the payoff is large. It tends to make you a more successful, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.